Mastering Firmware Development: A Complete Guide

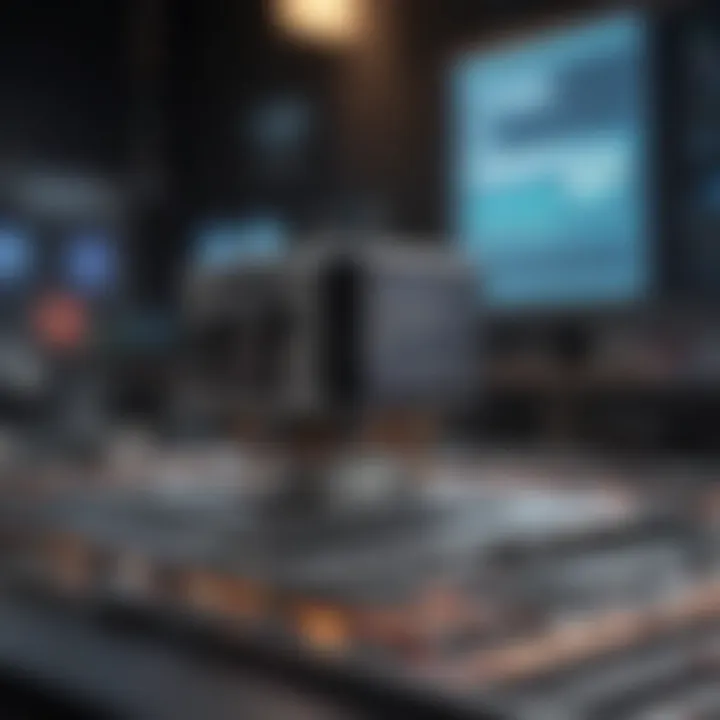
Intro
Firmware development is a specialized field that interacts with both hardware and software, creating a vital link in technologyβs chain. As devices grow more complex, the need for skilled firmware developers increases. This guide aims to provide a comprehensive understanding of firmware development, exploring its principles, applications, and best practices.
In modern technology, firmware is crucial for ensuring devices function as intended. Whether it is in embedded systems, consumer electronics, or industrial machinery, firmware controls the hardware and interfaces with software. Understanding this interplay is essential for anyone seeking to master firmware development. This article is an extensive resource designed for students, researchers, educators, and professionals who aim to deepen their knowledge and skills in firmware development.
Prelude to Firmware Development
Firmware development represents a crucial aspect of the ever-evolving technology landscape. It bridges the gap between hardware and software, ensuring that devices operate efficiently and respond accurately to user inputs. Understanding firmware is vital for anyone looking to delve into embedded systems, IoT devices, or other hardware-centric projects. This section elucidates the key components of firmware development, its significance, and the foundational knowledge essential for mastery in this field.
Definition and Scope
Firmware can be defined as a specialized class of software that is closely tied to hardware devices. It provides the necessary instructions for how the device communicates with other computer hardware. Essentially, firmware serves as the intermediary layer that allows hardware to perform its intended functions.
The scope of firmware development is broad and varies significantly between devices. For instance, the firmware in a simple household appliance might manage basic on/off functionality, while in complex devices like smartphones or medical equipment, firmware can control advanced operations, providing real-time processing and data analysis.
Key considerations in this realm include:
- Compatibility: Ensuring that firmware works seamlessly with the hardware it is designed for.
- Updates: Adapting firmware through updates to enhance functionality or improve security.
- Optimization: Tailoring firmware to balance performance with resource utilization, which is crucial in embedded systems where memory and processing power can be limited.
Historical Context
The concept of firmware has its roots in the early days of computing when hardware components were much less sophisticated than today. Initially, firmware was often hardwired into devices, requiring physical changes to update or alter the functionality of the hardware. With the advent of programmable devices, like microcontrollers, the nature of firmware started to shift, emphasizing flexibility and ease of updates.
During the 1980s and 1990s, as personal computing became widespread, firmware gained more attention due to its role in enhancing device capabilities. The rise of the Internet and connected devices further accelerated this trend, promoting the need for efficient firmware to handle various tasks, from simple data processing to complex networking functions.
The ongoing evolution of firmware reflects trends in technology, such as:
- Microcontroller advancements: With increased capabilities, modern microcontrollers support rich firmware programming environments.
- IoT expansion: The surge in Internet of Things (IoT) devices has necessitated a focus on secure, reliable firmware that can support connectivity and interaction.
Overall, a solid understanding of these elements provides a strong foundation to engage with firmware development more comprehensively. Emphasizing both the historical significance and the technical scope enhances the appreciation of its role in modern technology.
Key Concepts in Firmware Development
Understanding key concepts in firmware development is essential for anyone looking to navigate this specialized field. These concepts lay the groundwork for effective design, implementation, and maintenance of firmware. Skills in this area are growing increasingly important as modern technology becomes more intertwined with embedded systems. This section focuses on foundational topics such as microcontroller basics, embedded systems, and programming languages that are significant for firmware development.
Microcontroller Basics
Microcontrollers serve as the heart of many embedded systems. They are compact integrated circuits designed to govern specific tasks. Understanding the architecture of microcontrollers is crucial. Generally, they consist of a processor core, memory, and input/output peripherals. This arrangement allows the microcontroller to execute programs and interact with external devices efficiently.
Key components to note include:
- CPU (Central Processing Unit): Executes instructions and controls operations.
- RAM (Random Access Memory): Temporary data storage, which is volatile.
- ROM (Read-Only Memory): Permanent storage for firmware.
- I/O Ports: Interfaces for communication with other hardware.
Developers must grasp these building blocks to choose the right microcontroller for their projects, whether it involves simple functions or complex operations. Brands like STMicroelectronics and Microchip Technology provide a range of microcontrollers suitable for different applications, and familiarity with their specifications can significantly enhance project outcomes.
Embedded Systems Overview
Embedded systems are integral to numerous modern devices we encounter daily, from household appliances to automotive controls. An embedded system combines hardware and software tailored for a specific purpose. Understanding embedded systems involves recognizing their real-time constraints and limited resources.
Key features of embedded systems include:
- Real-Time Operation: The system must perform tasks within strict timing constraints.
- Resource Constraints: Limited processing power, memory, and storage capacity.
- Dedicated Functionality: Unlike general-purpose computers, they focus on singular tasks.
Familiarity with embedded systems opens up avenues in various industries, including automotive, healthcare, and consumer electronics. This increased demand for specialized embedded knowledge makes it a valuable skill set in todayβs job market.
Embedded and Assembly Language
Programming languages form the backbone of firmware development. Embedded C is widely used due to its efficiency in managing hardware resources. It allows for close interaction with hardware while providing higher-level abstractions than assembly language. Developers appreciate its portability across different microcontroller architectures.
Some advantages of using Embedded C in firmware development are:
- Portability: Write code that can run on various platforms with minimal changes.
- Efficient Memory Usage: Control over hardware resources transitions well into smaller devices.
- Rich Libraries: A wealth of libraries simplifies complex tasks and enhances functionality.
Assembly language, on the other hand, is more complex but offers unmatched control over the hardware. It translates directly to the machine code, meaning developers have the potential to optimize performance to the last detail. It is mainly used in performance-critical applications where every clock cycle matters.
In summary, understanding Embedded C and Assembly language is vital for those delving into firmware development. Each language has its unique advantages, making them suitable for different types of projects and requirements.
"A firm grasp of key concepts is critical for successful firmware development and can significantly impact the efficiency and performance of embedded systems."
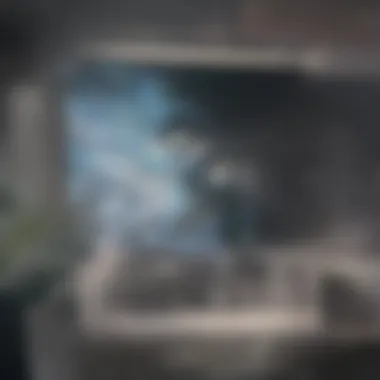
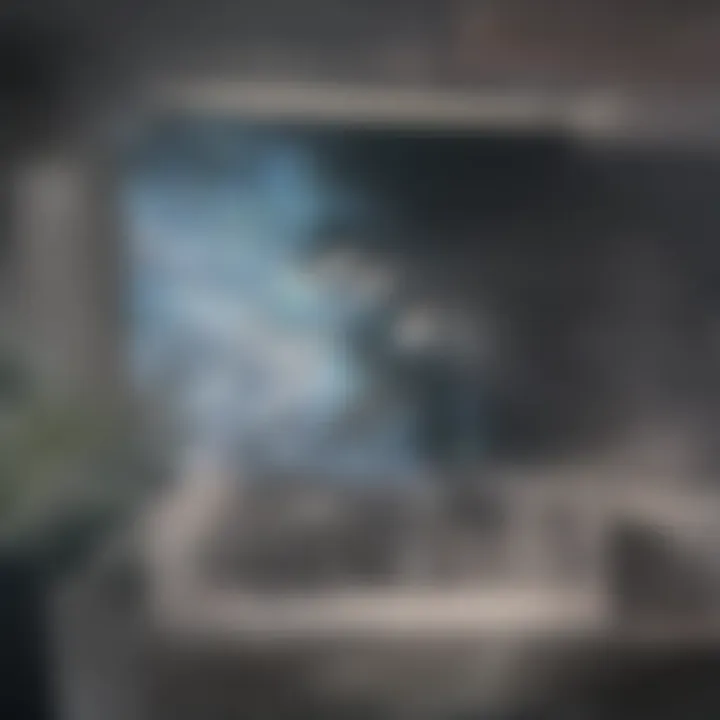
By exploring these key concepts, developers establish a strong foundation needed for advanced firmware development tasks.
Development Tools and Environment Setup
Setting up the right development tools and environment is crucial in the process of firmware development. These tools are the backbone that enables developers to write, test, and deploy firmware effectively. Without a well-structured environment, the process can become fragmented and inefficient. Each tool serves a specific role that aligns with the complexities and unique demands of firmware projects.
Integrated Development Environments (IDEs)
An Integrated Development Environment (IDE) is a software suite that consolidates basic tools required for software development. For firmware development, IDEs like Keil, MPLAB X, or Atmel Studio provide a unique set of functionalities. These environments typically include text editors, compilers, and debuggers in one interface, making it easier to manage code and execute complex tasks. The advantages of using an IDE include:
- Code Highlighting and Autocompletion: These features make coding faster and reduce errors.
- Built-In Debugging Tools: IDEs often come with debugging capabilities that allow tracking down issues in real-time.
- Version Control Integration: Developers can manage changes to the code efficiently through version control systems like Git.
Additionally, IDEs often have communities and extensive documentation. This resource can help developers resolve problems they encounter. Therefore, selecting an IDE that caters to your projectβs specific needs can lead to significant productivity gains.
Programming Languages Commonly Used
Certain programming languages dominate firmware development despite the wide-ranging options available. C, C++, and Assembly Language are among the most widely utilized. Each language has its advantages and suited applications:
- C: This language is the backbone of embedded systems due to its complexity management capabilities. It allows direct hardware manipulation, which is often necessary for firmware development.
- C++: While C remains the go-to for many, C++'s object-oriented features offer better abstraction and code reusability. This can be helpful for large projects.
- Assembly Language: Although less common for larger applications, Assembly language offers the most direct control over hardware. It can be essential for performance-critical sections of firmware.
When choosing a programming language, consider the specific hardware requirements and the functional goals of your project. The interaction between the programming language and the underlying hardware is crucial for optimized performance.
Hardware Toolchains and Debuggers
The hardware toolchain comprises all components involved in developing and testing firmware on a specific platform. It includes compilers, libraries, and linked tools that facilitate communication with the hardware. Debuggers are equally vital, providing insights into how the code operates in real time. Some notable aspects are:
- Compilers: These convert high-level code into machine code that the hardware can execute. Tools like GCC and IAR Embedded Workbench are common choices.
- Linkers: They help combine different modules of code into a single executable program.
- Debugging Interfaces: Earlier development only offered software debugging, but modern approaches include hardware tools such as JTAG or SWD that connect to the device directly, offering much deeper insights into the codeβs operation.
Selecting the right tools within the hardware toolchain can dramatically affect the efficiency of development and the reliability of the end product.
"A well-configured toolchain can be the difference between a project that meets deadlines and one that drags on indefinitely.β
By paying close attention to the tools and environment you choose for firmware development, you lay the groundwork for success in complex systems. Whether selecting an IDE, programming languages, or debugging tools, each choice should reflect the particular needs of the project.
The Firmware Development Process
The firmware development process is central to creating reliable and efficient embedded systems. It encompasses several critical phases that guide the transition from concept to creation. Understanding this process is essential for both novices and seasoned professionals. It ensures that the product meets its requirements while maintaining quality and performance. This section provides an overview of the elements involved, their benefits, and considerations during the development phase.
Project Planning and Requirements Gathering
Project planning is the foundation of any successful firmware development endeavor. It lays out the roadmap and aligns all stakeholders on the objectives. This phase involves defining the project scope, identifying stakeholders, and establishing clear requirements. It is crucial to engage with users and clients to understand their needs deeply.
Benefits of thorough project planning include:
- Reduced Risks: Identifying potential pitfalls early minimizes risks later in the process.
- Resource Allocation: Ensures the right resources are assigned to critical tasks.
- Timely Delivery: A well-structured plan helps keep the development schedule on track.
Gathering requirements requires careful attention to detail. Use various methods such as interviews, surveys, and workshops to gather information. Document all findings clearly to create a basis for the subsequent design stage.
Design and Architecture Considerations
Designing firmware involves a blend of creativity and technical considerations. The architecture must accommodate both the hardware and software constraints. Key factors in this stage include scalability, maintainability, and performance. Effective design helps foresee future changes and functionalities while ensuring the current needs are satisfied.
When designing firmware, consider:
- Modularity: Break the system into smaller components. This enhances maintainability.
- Efficiency: Optimize resource usage, especially in limited environments like microcontrollers.
- Compliance: Ensure that the architecture adheres to relevant standards and regulations.
A well-thought-out architecture leads to a smoother development process and easier updates in the future. For example, using a layered architecture can separate concerns and simplify modifications.
Implementation Strategies
The implementation phase is where the actual coding occurs. It requires translating design documents into functional code that adheres to the specified requirements. Choosing the right programming languages and tools is crucial. Many developers prefer Embedded C due to its efficiency and control over hardware.
Consider these strategies during implementation:
- Incremental Development: Allows for gradual integration and testing of features.
- Version Control: Use systems like Git for tracking changes and collaboration.
- Code Reviews: Regularly review code to catch errors early and improve code quality.
Incorporating testing within the implementation phase is essential. This includes unit tests to validate individual components before integrating them. Automated testing frameworks can streamline this process, ensuring quality at every step.
Remember: Effective communication among team members during this phase often contributes significantly to the success of the project.
By understanding and applying these principles of the firmware development process, developers can create robust and efficient firmware that meets ever-evolving market demands.
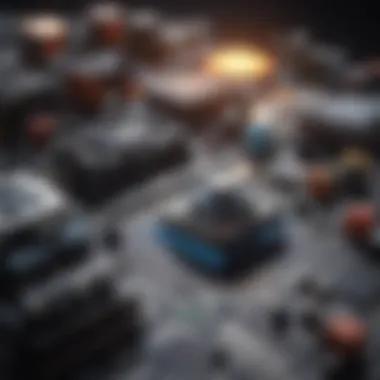
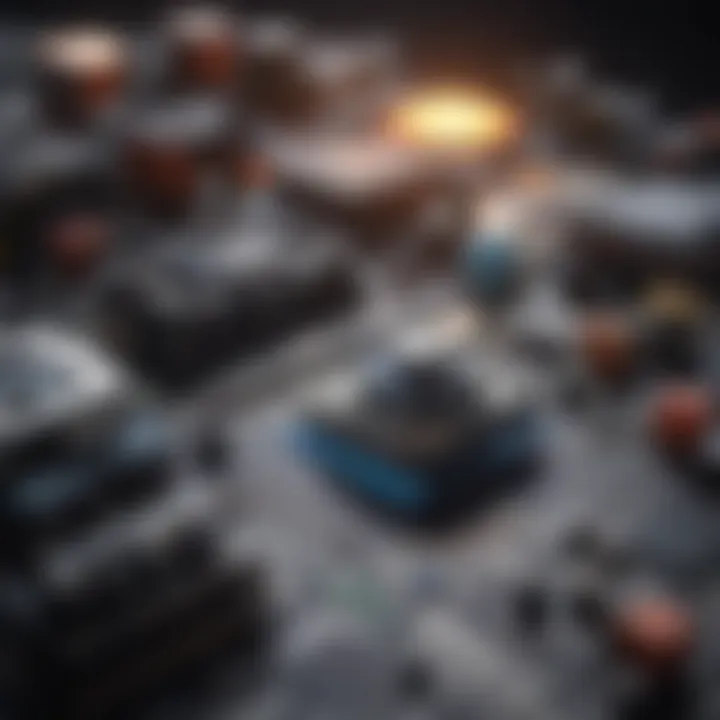
Testing and Validation
Testing and validation are critical components of firmware development, ensuring that the software performs as expected on the hardware it controls. These processes help identify errors or inconsistencies early in the development life cycle, significantly increasing the reliability of the firmware. Given the complex interplay between hardware and software, it is crucial to validate that firmware operates according to specified requirements.
Unit Testing in Firmware
Unit testing involves testing individual components or functions of the firmware independently, allowing developers to verify that each part operates correctly before integration. This method enhances code quality by ensuring that every function behaves as expected under predictable conditions.
- Isolation: Each unit is tested in isolation from the rest of the system. This is essential to prevent other components from affecting the outcome of tests.
- Test Cases: Automated test cases can be written to evaluate different scenarios for a function. Coverage can range from typical input values to edge cases that might cause errors.
- Frameworks: Utilizing frameworks such as Unity or Ceedling can simplify the process of running unit tests. They allow easy setup and accessing testing protocols.
Unit testing contributes to better maintainability of firmware. Bugs can surface earlier in the development cycle, and refactoring becomes less risky since unit tests help ensure existing functionality is preserved.
Integration Testing Techniques
Once unit testing is completed, integration testing comes into play. This process involves combining different units and testing their interactions, assuring the system works cohesively.
- Bottom-Up Approach: In this method, lower-level modules are tested first. Once they are verified, higher-level modules are integrated.
- Top-Down Approach: Here, the higher-level modules are tested first. Dummy components, called stubs, can simulate lower-level modules, allowing the system to execute.
- Incremental Integration: This technique tests components in increments, verifying functionality after each additional integration step.
Integration testing is vital for identifying issues in ways components work together, which could lead to unexpected behavior in the final product. Ensuring every part interacts smoothly is crucial for firmware's performance.
Debugging Tools and Methods
Debugging is an essential skill for firmware developers, as pinpointing the source of an issue can be quite complicated due to the interactions between hardware and software. The following are some tools and methods used in this process:
- In-Circuit Emulators (ICE): These tools allow developers to test the firmware in real-time on the hardware. They provide insights into how the firmware interacts with the microcontroller.
- JTAG Debuggers: Joint Test Action Group (JTAG) interfaces offer a standard for debugging devices, allowing low-level access to the system for detailed analysis.
- Print Debugging: While not always feasible in production environments, using print statements can provide insights into the code execution flow.
Even with the best planning and testing strategies, unexpected issues will arise during the firmware development. A systematic approach to debugging will facilitate identifying root causes and help ensure the final product is reliable.
"Testing and debugging are vital for firmware development as they minimize the risk of failure and enhance product quality."
Ultimately, a disciplined approach to testing and validation allows developers to create robust firmware, fostering confidence in its deployment across a variety of systems.
Deployment and Maintenance of Firmware
The deployment and maintenance of firmware represent critical phases in the overall lifecycle of embedded systems. Correctly navigating these processes not only ensures that devices function as intended but also contributes to system longevity and security. Firmware, by nature, acts as the bridge between hardware and software, making its management a crucial skill for firmware developers.
Once development is complete, the deployment phase begins, which involves transferring the firmware onto the target device. This process may include various mechanisms for updating existing firmware on devices already in use. Several elements play a role in the deployment phase. First, factors like update frequency, size, and the associated risks must be considered. Effective planning can prevent interruptions in service and avoid potential failures in hardware operation.
Moreover, the maintenance aspect of firmware ensures that devices stay secure and functional over time. Given the rapid advancement in technology and security threats, developers must prioritize updates to address vulnerabilities. Ongoing maintenance involves collecting performance metrics, monitoring system integrity, and deploying necessary updates promptly. A proactive maintenance strategy is essential to extend the functional and operational lifespan of devices.
Firmware Update Mechanisms
Firmware update mechanisms are vital for keeping devices in peak operating condition. These mechanisms can vary widely, but they generally fall into two main categories: manual updates and automatic updates.
- Manual Updates: This method requires user intervention to download and install updates. Users must be aware of new firmware versions and follow specific instructions, which can lead to inconsistencies due to human error.
- Automatic Updates: This approach allows devices to check for updates and install them automatically without user interaction. Automatic updates reduce the likelihood of outdated firmware but can introduce problems if not managed correctly.
The implementation of secure update protocols is another consideration. Strategies such as over-the-air (OTA) updates allow developers to send new firmware to devices remotely, which can simplify the updating process. Security measures like digital signatures and verification checks are vital here to confirm the integrity and authenticity of the firmware being installed.
In some cases, the firmware update mechanism may need to support rollback capabilities. This allows a device to revert to a previous version of the firmware if a new version causes issues. The consideration of adequate testing and verification procedures before deploying updates is essential to maintain device reliability.
Monitoring and Performance Metrics
Monitoring is a fundamental aspect of firmware maintenance. Effective monitoring allows developers and operators to gauge system performance and identify issues proactively. Implementing performance metrics provides quantitative data that can inform better decision-making regarding firmware updates and system optimizations.
Common performance metrics can include:
- CPU Usage: Indicates how much processing power the firmware uses. High CPU utilization may suggest inefficiencies or bugs in the code.
- Memory Usage: This metric reveals how much RAM the firmware consumes, which is crucial for systems with limited resources.
- Battery Life: Particularly in portable devices, monitoring battery consumption can inform firmware improvements aimed at extending device usage time.
"Monitoring and analyzing performance metrics allows developers to optimize firmware actively, enhancing both reliability and user experience."
In addition to traditional metrics, real-time monitoring can provide insights into device operation and help troubleshoot issues as they arise. By implementing automated monitoring solutions, developers can obtain timely data that supports more responsive maintenance actions. Thus, effective monitoring and performance metric strategies are vital for creating a sustainable firmware lifecycle.
Common Challenges in Firmware Development
Firmware development presents a unique set of challenges that can impact both the quality of the product and the efficiency of the development process. Understanding these challenges is crucial for any developer looking to create reliable and effective firmware. Overcoming such problems not only improves the final product but also streamlines future development efforts. The focus here will be on two significant areas: debugging complex firmware issues and addressing hardware and software compatibility issues. Navigating these challenges often requires a mix of technical skills, strategic planning, and persistent problem-solving.
Debugging Complex Firmware Issues
Debugging in firmware development can be exceptionally challenging due to the close interplay between hardware and software. Unlike traditional software debugging, which often runs in isolated environments, firmware operates directly on physical hardware. This means that problems can manifest in numerous, sometimes unexpected ways.
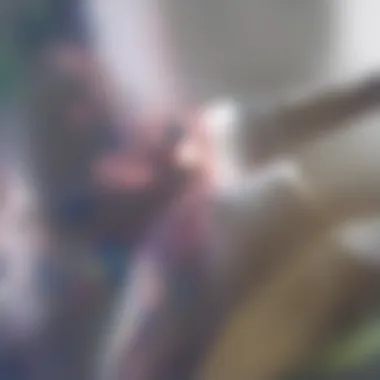
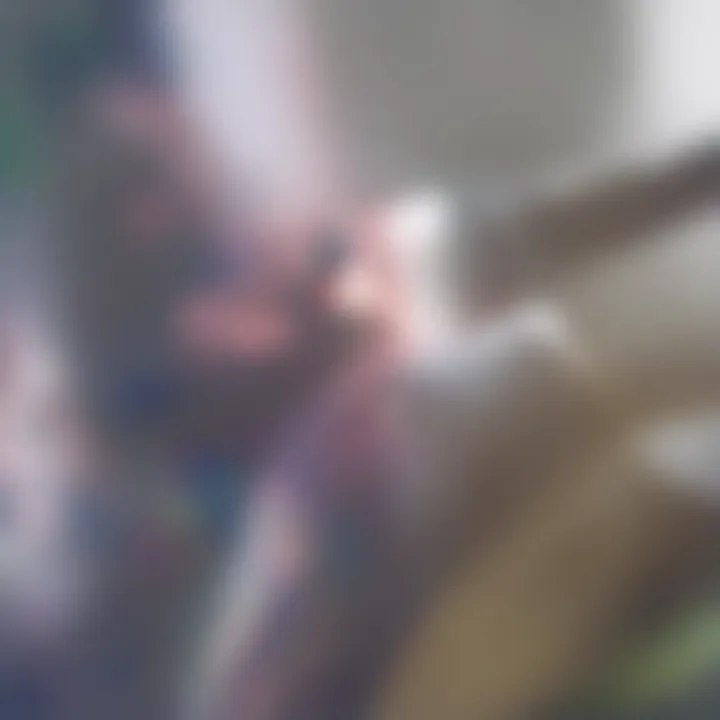
Common issues include memory leaks, race conditions, and peripheral glitches. These problems are difficult to identify since they may not always produce clear error messages or symptoms. Debugging tools can include traditional software debugging via IDEs, but developers often rely on hardware-based techniques such as JTAG or UART.
"Debugging firmware requires both intuition and technical know-how, as many problems stem from hardware interactions that may not be easily visible to the developer."
Effective strategies include:
- Incremental Testing: Develop and test firmware in manageable sections to isolate issues quickly.
- Logging: Implement logging at various points in the firmware to track the state and values during execution.
- Simulators: Use simulators to recreate environments and test without needing the actual hardware.
By applying thorough testing protocols and leveraging effective tools, developers can reduce the potential for severe bugs and enhance the firmwareβs reliability.
Hardware and Software Compatibility Issues
Another prevalent challenge in firmware development is achieving compatibility between hardware and software components. As technology evolves, the integration of various components may lead to misalignment. Each hardware component has its specific requirements that must be addressed in the firmware. Failure to do so can result in significant functionality issues, impacting the overall performance of the device.
For instance, firmware designed for an older version of a microcontroller may not perform correctly on newer iterations due to changes in architecture or included features. Moreover, different peripherals may have their unique communication protocols or timing requirements, leading to further complications.
To combat these challenges, developers should consider:
- Version Control: Maintain versions of both hardware and firmware to track compatibility over time.
- Documentation: Keep up-to-date documentation regarding hardware specifications and software requirements.
- Testing with Variants: Test firmware against different hardware configurations to ensure robustness.
Understanding these challenges will empower developers with the analytical tools and strategies needed to create effective and reliable firmware solutions. This knowledge can enhance problem-solving abilities and lead to improved development practices.
Future Trends in Firmware Development
The technology landscape is ever-evolving, and firmware development is not immune to these changes. Understanding the future trends in this field is essential for developers, students, and researchers alike. Emphasizing these trends assists in anticipating shifts in project requirements and emerging technologies. Keeping abreast of these trends ensures that developers remain relevant in a competitive job market.
The Rise of IoT and Connected Devices
The Internet of Things (IoT) is arguably one of the most significant trends impacting firmware development. IoT encompasses a wide range of connected devices, from everyday household items to advanced industrial machinery. As these devices proliferate, the demand for efficient, reliable firmware grows.
- Complexity of Systems: IoT devices often involve multiple hardware components working together. Developers must be adept at managing this complexity to ensure seamless operation.
- Security Concerns: With more devices connected to the internet, security becomes paramount. Firmware developers must integrate security practices in their development process to safeguard against attacks.
- Real-time Data Processing: Many IoT applications require real-time data processing. The firmware must support fast response times to be effective.
The rise of IoT also leads to unique challenges in development, such as varying standards and protocols. Adapting to these changes requires continuous learning and adjustment of development practices.
"The future of IoT heavily relies on robust firmware capable of handling real-time data seamlessly and securely."
Advancements in Machine Learning Applications
Machine learning is another area reshaping firmware development. As businesses adopt machine learning, the firmware becomes integral to executing algorithms directly in devices. This evolution brings several important aspects:
- In-device Processing: Many applications now perform computations on-device rather than relying solely on server resources. This change necessitates more sophisticated firmware capable of managing these tasks efficiently.
- Adaptive Firmware: With machine learning, firmware can be designed to adapt its behaviour based on usage patterns. This adaptability allows devices to optimize performance in real-time.
- User Personalization: Firmware powered by machine learning enhances personalization for end-users. This ability to learn from user interactions can vastly improve the user experience.
Incorporating machine learning techniques into firmware development poses challenges around performance and resource management. Developers must balance functionality with the constraints of the device they are programming.
As the field of firmware development progresses, these trends will play a pivotal role in shaping the competencies required for success. To thrive, developers should invest time in understanding IoT technologies and machine learning concepts.
Ending and Recommendations
In the realm of firmware development, the conclusions drawn from comprehensive study and practice are pivotal in shaping future endeavors. This article outlines critical components essential for grasping the multilayered nature of firmware. From its theoretical foundations to practical applications, understanding these elements enables developers to navigate potential pitfalls while harnessing opportunities.
Recommendations encapsulate both best practices and suggestions for continuous advancement. New developers should not only familiarize themselves with hardware and embedded programming but also embrace iterative learning and effective problem-solving techniques. Encouragement towards collaboration with seasoned professionals can provide invaluable insights, leading to an accelerated learning curve.
Key elements worthy of note include:
- The commitment to perennial learning in emerging technologies.
- Participation in forums such as Reddit, where troubleshooting and innovation are frequent topic.
- Engagement in projects that test theoretical knowledge against real-world scenarios.
Benefits of Following Recommendations:
- Enhanced skills through exposure to diverse projects.
- Increased ability to adapt to advancements in firmware technologies.
- Opportunities to contribute to community-driven initiatives which benefit both personal and collective growth.
"Success in firmware development is not only about technical skills but also about a mindset willing to learn and adapt."
Best Practices for New Developers
To position themselves for success, new developers should integrate best practices into their workflow. These practices foster efficiency, quality, and adaptability, essential attributes in firmware development. Here are some guidelines:
- Understand Hardware Limitations: Grasp the nuances of the hardware being programmed. It helps to align software capabilities with device constraints.
- Version Control Utilization: Adopt tools like Git to maintain code versions and collaborate seamlessly with teams.
- Adopt Modular Coding Practices: Write code in small, manageable blocks. This facilitates debugging, testing, and future modifications.
- Thorough Documentation: Documenting each phase of development promotes clarity. Clear documentation can aid not only the developer but also teams involved across the project lifecycle.
- Seek Feedback: Regular reviews of code by peers help refine skills and promote knowledge-sharing.
These practices not only contribute to the development process but also foster mental discipline, which is vital in consistently delivering quality work.
Resources for Further Learning
For further exploration, several resources stand out:
- Wikipedia: A vast repository of knowledge, especially the pages on Firmware and Embedded Systems.
- Britannica: Provides reliable articles on embedded software development, useful for foundational understanding.
- Online Communities: Forums on platforms like Reddit, where discussions on recent advancements and troubleshooting tips can provide practical insights.
- Books and Courses: Seek out influential books such as "Embedded C Programming and the Atmel AVR" for practical algorithms and real-world applications.
Building a solid foundation requires an investment of time in quality learning materials and practical experience. Each resource serves a specific purpose and guides through various challenges encountered along the way.